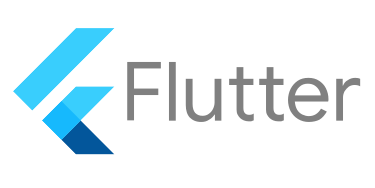
Implementing Google Fonts in Flutter: From App-wide Themes to Individual Widgets
Introduction
Flutter provides easy access to over 800 fonts through the Google Fonts package. In this post, let’s explore how to implement Google Fonts in Flutter applications, from basic implementation to applying it across your app’s theme.
Setting Up Google Fonts Package
1. Adding Dependencies
First, add the google_fonts package to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
google_fonts: ^6.2.1
Then run the following command in your terminal:
flutter pub get
Alternatively, install directly from the terminal:
flutter pub add google_fonts
2. Basic Usage
Let’s look at the most basic way to use Google Fonts:
import 'package:google_fonts/google_fonts.dart';
class MyWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Text(
'Hello, Flutter!',
style: GoogleFonts.notoSans(
fontSize: 24,
fontWeight: FontWeight.bold,
color: Colors.blue,
),
);
}
}
Applying Google Fonts to App-wide Theme
1. ThemeData Setup
Here’s how to apply Google Fonts to your app’s entire theme:
import 'package:flutter/material.dart';
import 'package:google_fonts/google_fonts.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Google Fonts Demo',
theme: ThemeData(
// Set default text theme
textTheme: GoogleFonts.notoSansTextTheme(
Theme.of(context).textTheme,
),
// Use different font for heading style
primaryTextTheme: GoogleFonts.notoSerifTextTheme(
Theme.of(context).primaryTextTheme,
),
),
home: MyHomePage(),
);
}
}
2. Custom Theme Setup
For more detailed styling, you can configure like this:
ThemeData(
textTheme: TextTheme(
displayLarge: GoogleFonts.notoSans(
fontSize: 32,
fontWeight: FontWeight.bold,
color: Colors.black87,
),
bodyLarge: GoogleFonts.notoSans(
fontSize: 16,
color: Colors.black87,
),
titleMedium: GoogleFonts.notoSerif(
fontSize: 20,
fontWeight: FontWeight.w500,
color: Colors.black,
),
),
)
Alternative Implementations
1. Creating a Blog Style Widget
class BlogPostWidget extends StatelessWidget {
final String title;
final String content;
const BlogPostWidget({
Key? key,
required this.title,
required this.content,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
title,
style: GoogleFonts.notoSerif(
fontSize: 24,
fontWeight: FontWeight.bold,
height: 1.5,
),
),
SizedBox(height: 16),
Text(
content,
style: GoogleFonts.notoSans(
fontSize: 16,
height: 1.8,
letterSpacing: 0.5,
),
),
],
);
}
}
2. Reusing Font Styles
You can define frequently used styles as constants for reuse:
class AppStyles {
static final headerStyle = GoogleFonts.notoSerif(
fontSize: 24,
fontWeight: FontWeight.bold,
height: 1.5,
);
static final bodyStyle = GoogleFonts.notoSans(
fontSize: 16,
height: 1.8,
letterSpacing: 0.5,
);
static final captionStyle = GoogleFonts.notoSans(
fontSize: 14,
color: Colors.grey[600],
height: 1.5,
);
}
// Usage example
Text('Title', style: AppStyles.headerStyle);
Performance Optimization Tips
1. Font Caching
Google Fonts caches fonts by default, but you can also preload them for offline use:
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
bool _fontsLoaded = false;
@override
void initState() {
super.initState();
_loadFonts();
}
Future<void> _loadFonts() async {
await GoogleFonts.pendingFonts([
GoogleFonts.notoSans(),
GoogleFonts.notoSerif(),
]);
setState(() {
_fontsLoaded = true;
});
}
@override
Widget build(BuildContext context) {
if (!_fontsLoaded) {
return CircularProgressIndicator();
}
// Rest of the app build logic...
}
}
2. Memory Usage Optimization
When font styles don’t change frequently, define them as constants to optimize memory usage:
// Good example
static final headerStyle = GoogleFonts.notoSerif();
// Example to avoid (inside build method)
Text('Title', style: GoogleFonts.notoSerif()); // Creates new TextStyle object each time
Summary
Google Fonts in Flutter offers easy implementation of various fonts. It provides flexible usage options, from app-wide themes to individual widgets, along with various methods for performance optimization.
Key points to remember:
- Use
GoogleFonts.xxxTextTheme()
for app-wide theme application - Apply
GoogleFonts.xxx()
for individual styling - Define frequently used styles as constants for reuse
- Preload required fonts for offline use