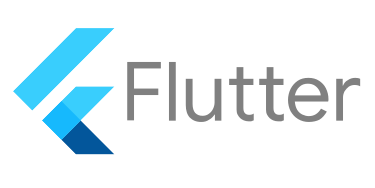
Responsive Design in Flutter: Handling Device Sizes
One of the most crucial aspects of mobile app development is adapting to various device sizes. When developing cross-platform apps with Flutter, you need to consider all screen sizes, from smartphones to tablets and desktops. Today, we’ll explore in detail how to check and handle device sizes in Flutter.
Understanding Device Size Units
The Difference Between Pixels (px) and DP
First, we need to understand the difference between pixels (px) and DP (Density-independent Pixels).
A pixel is the smallest unit that makes up a screen, representing an actual physical point on the display. However, since devices have different screen sizes and resolutions, designing UI with pixel units can result in inconsistent sizes across devices.
DP is a unit introduced by Android to solve this problem. 1dp equals 1 pixel on a 160dpi screen. For example, on a 320dpi screen, 1dp equals 2 pixels. In Flutter, these are called logical pixels, helping maintain consistent sizes in cross-platform development.
Checking Screen Size in Flutter
Using MediaQuery
MediaQuery is the most basic way to handle screen sizes in Flutter. MediaQuery is a widget that provides various information about the device running the app, including screen size, orientation, system font size, brightness settings, and more.
Particularly, you can get MediaQueryData from the current screen’s context using MediaQuery.of(context), which allows you to easily obtain necessary information as shown below:
class ResponsiveWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Get screen size information
final size = MediaQuery.of(context).size;
final width = size.width;
final height = size.height;
// Check screen orientation
final isPortrait = MediaQuery.of(context).orientation == Orientation.portrait;
// Check device pixel ratio
final devicePixelRatio = MediaQuery.of(context).devicePixelRatio;
return Container(
// Widget construction based on screen size
);
}
}
Utilizing LayoutBuilder
LayoutBuilder allows you to create different layouts based on the parent widget’s constraints. The key difference from MediaQuery is that it works based on the parent widget’s size constraints rather than the entire screen size.
For example, if you use LayoutBuilder inside a Container that takes up half the screen, constraints.maxWidth will be half the screen width. This characteristic makes it particularly useful when you want to apply responsive design only within specific areas.
The example below shows how to return different layouts based on screen width:
class AdaptiveLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth < 600) {
return MobileLayout();
} else if (constraints.maxWidth < 900) {
return TabletLayout();
} else {
return DesktopLayout();
}
},
);
}
}
Determining Device Type
In real development, there are times when you need to distinguish device types beyond just size. Following Material Design guidelines, devices are generally categorized as mobile when under 600dp, tablet between 600dp and 900dp, and desktop at 900dp and above.
Creating a utility class that can easily determine device type using these standards allows you to implement responsive design consistently throughout your app. Here’s an example class with static methods for simple device type checking:
class DeviceType {
static bool isMobile(BuildContext context) {
return MediaQuery.of(context).size.width < 600;
}
static bool isTablet(BuildContext context) {
return MediaQuery.of(context).size.width >= 600 &&
MediaQuery.of(context).size.width < 900;
}
static bool isDesktop(BuildContext context) {
return MediaQuery.of(context).size.width >= 900;
}
}
Implementing Responsive Design
1. Basic Responsive Layout
Grid layouts are one of the most commonly used patterns when implementing responsive design. By dynamically adjusting the number of grid columns based on screen width, you can naturally adapt to various screen sizes.
The example below shows how to calculate grid column count by dividing screen width by 200dp units. This way, the number of columns naturally increases as the screen gets wider. Setting a minimum column count of 2 ensures appropriate layout even on very narrow screens.
class ResponsiveLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: LayoutBuilder(
builder: (context, constraints) {
double maxWidth = constraints.maxWidth;
// Adjust grid column count
int crossAxisCount = maxWidth ~/ 200;
if (crossAxisCount < 2) crossAxisCount = 2;
return GridView.builder(
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: crossAxisCount,
childAspectRatio: 1.5,
),
itemBuilder: (context, index) {
return GridItem();
},
);
},
),
),
);
}
}
2. Handling Screen Orientation
On mobile devices, you often need to provide different layouts for different screen orientations (portrait/landscape). OrientationBuilder makes it easy to detect and respond to orientation changes.
In the example below, we’ve implemented a 2-column grid for portrait mode and a 3-column grid for landscape mode. This pattern is particularly useful when displaying grid-based content like galleries or product lists.
class OrientationResponsiveWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return OrientationBuilder(
builder: (context, orientation) {
return GridView.count(
crossAxisCount: orientation == Orientation.portrait ? 2 : 3,
children: List.generate(6, (index) {
return Card(
child: Center(
child: Text('Item $index'),
),
);
}),
);
},
);
}
}
Practical Example: Responsive Dashboard
In real projects, you often need to provide completely different layouts based on device characteristics, beyond just handling size or orientation. A dashboard is a prime example that demonstrates this situation well.
The example below categorizes layouts into three types based on screen width:
- Desktop (1200dp or more): 3-panel layout showing sidebar, main content, and details
- Tablet (800dp-1200dp): 2-panel layout showing sidebar and main content
- Mobile (under 800dp): Single column layout with header and main content
You can fine-tune the proportions of each area using the flex property of the Expanded widget.
class DashboardScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth >= 1200) {
// Desktop layout
return Row(
children: [
Expanded(
flex: 2,
child: DashboardSidebar(),
),
Expanded(
flex: 5,
child: DashboardContent(),
),
Expanded(
flex: 2,
child: DashboardDetails(),
),
],
);
} else if (constraints.maxWidth >= 800) {
// Tablet layout
return Row(
children: [
Expanded(
flex: 1,
child: DashboardSidebar(),
),
Expanded(
flex: 2,
child: DashboardContent(),
),
],
);
} else {
// Mobile layout
return Column(
children: [
DashboardHeader(),
Expanded(
child: DashboardContent(),
),
],
);
}
},
);
}
}
Performance Optimization Tips
When implementing responsive design, performance should also be considered.
-
Preventing Unnecessary Rebuilds
- Avoid complex calculations in MediaQuery or LayoutBuilder callbacks
- Update only necessary parts through state management
-
Utilizing Caching
- Cache frequently used size calculation results
- Provide images at resolutions appropriate for device size
Conclusion
Implementing responsive design in Flutter isn’t as difficult as it might seem. By properly utilizing MediaQuery and LayoutBuilder and understanding device characteristics, you can create apps that work well across all screen sizes.
The unit system is often the most confusing part for web developers transitioning to Flutter, but development becomes much smoother once you get used to using dp instead of px.
As new devices continue to be released, mastering these responsive design patterns will enable you to skillfully adapt to any device that comes along.