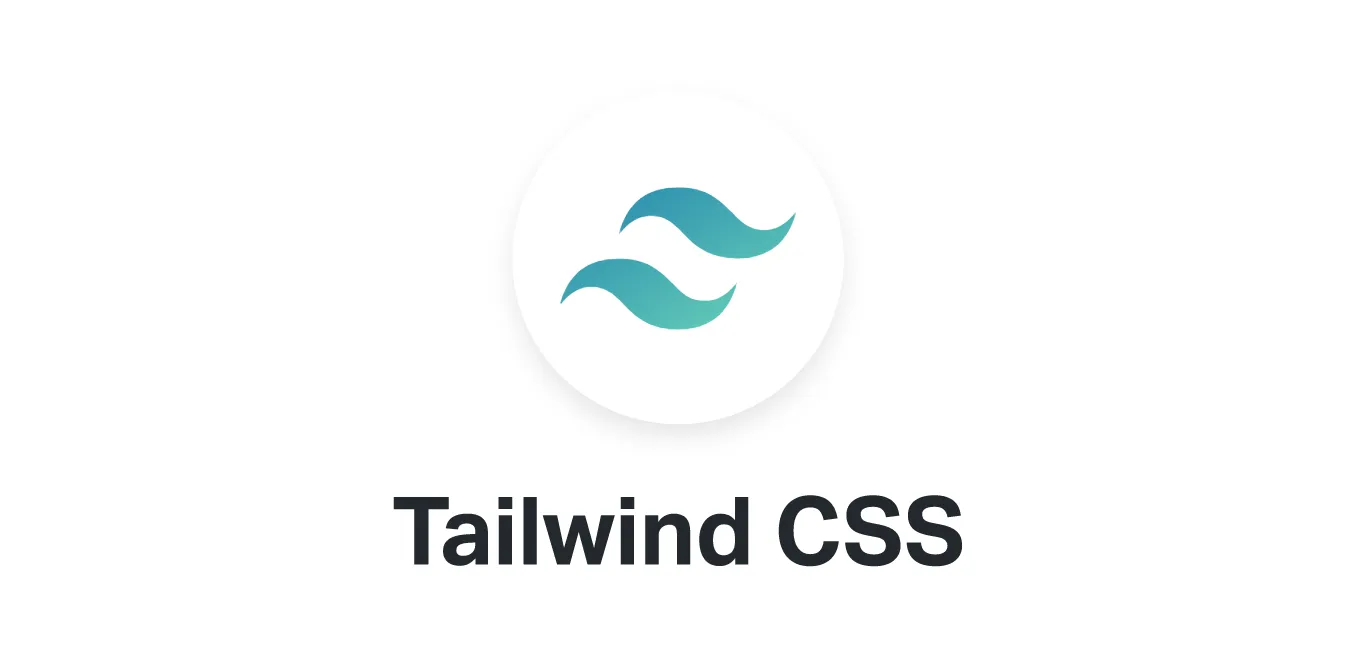
Tailwind CSS vs styled-components
Recently, whether by chance or intentionally, I had the opportunity to compare and contrast two different styling approaches. I used styled-components for our company website development and Tailwind CSS for my personal project, which gave me insights into their respective strengths and weaknesses.
In web development, CSS-in-JS and utility-first CSS represent different philosophical approaches and methodologies. In this article, I’ll dive deep into comparing two popular styling solutions: Tailwind CSS and styled-components.
Basic Concept Comparison
Tailwind CSS
Tailwind CSS is a CSS framework that adopts a utility-first approach. It operates by combining predefined classes to compose styles.
// Tailwind CSS example
function Button({ children }) {
return (
<button className="px-4 py-2 bg-blue-500 text-white rounded-md hover:bg-blue-600 transition-colors">
{children}
</button>
);
}
styled-components
styled-components is a CSS-in-JS library that allows you to write CSS directly within your JavaScript code.
// styled-components example
const Button = styled.button`
padding: 0.5rem 1rem;
background-color: #3b82f6;
color: white;
border-radius: 0.375rem;
transition: background-color 0.2s;
&:hover {
background-color: #2563eb;
}
`;
Development Productivity Comparison
The main reason I chose Tailwind CSS for my personal project was its rapid development capability. Since it was a personal project that didn’t require complex styling, just needing quick development, this factor heavily influenced my decision to go with Tailwind CSS.
On the other hand, I used styled-components for the company website because I needed to focus more on reusability and maintainability.
Advantages of Tailwind CSS
-
Rapid Prototyping
- Instant style application without worrying about class names
- Fast development with editor autocompletion
- Consistent styling within the configured design system
-
Low Learning Curve
- Easy to apply with CSS knowledge
- Intuitive class naming
- Well-documented with easily accessible references
-
Bundle Size Optimization
- Automatic removal of unused styles
- Minimized style declaration redundancy
Advantages of styled-components
-
Easy Dynamic Styling
const Button = styled.button` background-color: ${(props) => (props.primary ? "#3b82f6" : "#grey")}; color: ${(props) => (props.primary ? "white" : "black")}; `;
-
Component-Centered Development
- Encapsulation of styles and logic
- Highly reusable component creation
- No CSS collision concerns
-
TypeScript Integration
- Type safety
- Type checking for style changes through props
Real Development Scenario Comparison
Scenario 1: Developing a Responsive Card Component
[Previous code examples remain the same]
Development Time Comparison
-
Initial Setup
- Tailwind CSS: Configuration file creation and plugin installation needed (15-30 minutes)
- styled-components: Ready to use after npm installation (about 5 minutes)
-
Component Development
- Tailwind CSS: Quick development with class combinations (10-15 minutes)
- styled-components: Component separation and style definition required (20-25 minutes)
-
Maintenance
- Tailwind CSS: Readability management needed due to potentially long class names
- styled-components: Easy maintenance with clear component structure
Performance and Development Experience
Tailwind CSS uses a JIT (Just-In-Time) compilation system to generate only the styles actually used. This optimizes the final bundle size by removing unnecessary CSS code. It delivers excellent browser performance as no additional JavaScript execution is required at runtime. The browser’s cache mechanism can be effectively utilized, enabling faster loading on repeat visits. However, HTML files may become slightly larger due to multiple class names, and build processes might take additional time.
styled-components specializes in dynamic styling. It allows easy style changes based on props or state, and CSS code is automatically split and managed by component. However, this flexibility comes with a minor performance cost. Since styles are generated at runtime using JavaScript, there might be initial rendering delays, and browsers need to perform additional work.
Project-Specific Selection Criteria
Each technology’s selection largely depends on project characteristics and requirements. Tailwind CSS is particularly useful for rapid development and prototyping. Using predefined utility classes enables consistent design system implementation and makes it easy for teams to follow the same style guide. Its excellent performance can be a significant advantage in projects expecting high traffic.
styled-components is suitable for projects requiring complex dynamic styling. When styles need to change frequently based on user interactions or state, controlling styles through props is very convenient. For projects where TypeScript integration is important, styled-components’ type safety provides significant benefits. Its component-centered approach can also be advantageous when creating many reusable components.
Hybrid Approach Possibility
A hybrid approach combining both technologies is possible. You can utilize Tailwind CSS’s utility classes alongside styled-components’ dynamic styling, leveraging each technology’s strengths as needed. For example, implementing basic layouts and responsive design with Tailwind CSS while creating components requiring complex interactions with styled-components. To be honest, I haven’t tried this approach thoroughly yet, but I understand it’s a possibility.
const StyledCard = styled.div.attrs({
className: "rounded-lg shadow-lg",
})`
${(props) => props.customStyle}
`;
function Card({ customStyle }) {
return (
<StyledCard customStyle={customStyle}>
<div className="p-4">
<h2 className="text-xl font-bold">Title</h2>
</div>
</StyledCard>
);
}
Conclusion
Both technologies have their strengths and weaknesses, and the choice depends on project requirements and team preferences. Tailwind CSS excels in rapid development and consistent design system implementation, while styled-components shines in dynamic styling and component reusability.
Recent trends show Tailwind CSS’s rising popularity, particularly noted for its easy Next.js integration and excellent performance. However, this shouldn’t be the absolute criterion; the choice should consider project characteristics and team capabilities.