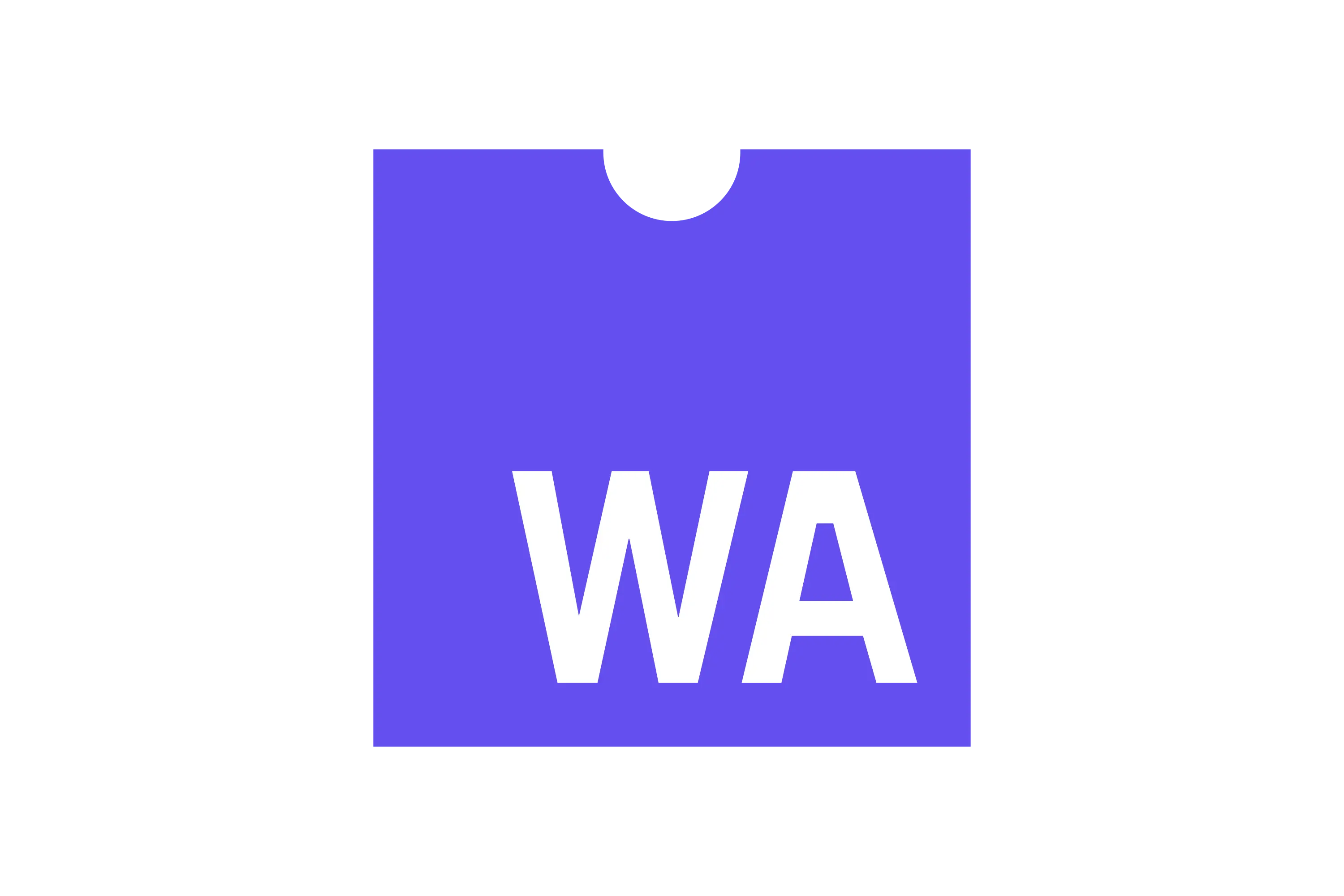
WebAssembly: The Future of Web Development
WebAssembly (Wasm) is a low-level bytecode that runs in the web browser, serving as a compilation target for various programming languages. Languages like Rust, AssemblyScript (similar to TypeScript), and Emscripten (C/C++) can be compiled to Wasm.
Flutter has also recently added support for WebAssembly builds in its latest updates.
History of WebAssembly
WebAssembly began as a W3C project in 2015, developed to improve web performance. It was initially proposed as an alternative to overcome JavaScript’s limitations and was designed to efficiently execute code written in various languages on the web.
The evolution of WebAssembly includes:
- 2015: Google, Microsoft, Mozilla, and Apple jointly announce the WebAssembly project
- 2017: First version (MVP) release and major browser support begins
- 2019: Approved as a W3C standard
- 2020: SIMD (Single Instruction Multiple Data) support added
- 2021: Garbage collection and JavaScript object reference features introduced
- 2022: Enhanced Web GPU integration
- 2023: Multithreading support and Exception Handling features added
Key Features of WebAssembly
1. Fast Performance
Wasm is provided in binary format, loading and executing faster than text-based JavaScript. Key performance factors include:
- Optimized Binary Format: Wasm binaries are provided in a format directly executable by browsers
- AOT (Ahead-of-Time) Compilation: Compiled to native code before execution for improved performance
- SIMD Operations Support: Enables parallel processing through vector operations
- Low Memory Overhead: Efficient memory management and garbage collection
Performance comparison example (Fibonacci sequence calculation):
// JavaScript implementation
function fibJS(n) {
if (n <= 1) return n;
return fibJS(n - 1) + fibJS(n - 2);
}
// Rust version compiled to Wasm
#[no_mangle]
pub fn fibWasm(n: i32) -> i32 {
if n <= 1 { return n; }
fibWasm(n - 1) + fibWasm(n - 2)
}
Execution time comparison (n=40):
- JavaScript: ~1500ms
- WebAssembly: ~300ms
2. Browser Compatibility
Supported by all major browsers and offers good interoperability with JavaScript.
// Loading Wasm module
const wasmInstance = await WebAssembly.instantiateStreaming(
fetch("example.wasm"),
{
env: {
consoleLog: (arg) => console.log(arg),
},
}
);
// Calling Wasm function from JavaScript
const result = wasmInstance.instance.exports.calculateSum(10, 20);
3. Security
WebAssembly’s security model features:
- Memory Isolation: Each Wasm module has its own isolated linear memory space
- Type Safety: All memory accesses and function calls undergo type checking
- Sandboxing: Only APIs provided by the host environment can be used
- CORS Policy Compliance: Follows web security policies
Security example code:
// Rust's memory safety is maintained in Wasm
pub fn safe_memory_access(arr: &[u32], index: usize) -> Option<u32> {
arr.get(index).copied()
}
4. Portability
Supports various platforms:
- Web Browsers: Chrome, Firefox, Safari, Edge
- Serverless Environments: AWS Lambda, Cloudflare Workers
- Edge Computing: Fastly Compute@Edge
- Desktop Applications: Tauri, Electron
- Mobile Platforms: React Native, Flutter
WebAssembly Use Cases
Game Development
Example of web game development using Unity:
// Unity WebGL build and Wasm integration
const unityInstance = await createUnityInstance(canvas, config, (progress) => {
progressBar.style.width = `${100 * progress}%`;
});
// Calling Unity function from JavaScript
unityInstance.SendMessage("GameController", "StartGame");
Real-world examples:
- Figma: Uses Wasm for vector graphics processing
- Google Earth: Ported 3D rendering engine to Wasm
- AutoCAD Web: Implemented CAD engine in Wasm
Data Visualization
Example of large-scale data processing combining D3.js and Wasm:
// Data processing logic implemented in Rust
#[wasm_bindgen]
pub fn process_data(data: &[f64]) -> Vec<f64> {
data.par_iter()
.map(|&x| x.powf(2.0) * 100.0)
.collect()
}
Machine Learning
Example of TensorFlow.js and Wasm integration:
// Using TensorFlow.js with Wasm backend
await tf.setBackend("wasm");
const model = await tf.loadGraphModel("model.json");
const prediction = model.predict(tf.tensor2d([[1, 2, 3, 4]]));
Multimedia Processing
Example of FFmpeg ported to Wasm:
const ffmpeg = createFFmpeg({ log: true });
await ffmpeg.load();
await ffmpeg.write("input.mp4", videoFile);
await ffmpeg.run("-i", "input.mp4", "output.gif");
const data = ffmpeg.read("output.gif");
WebAssembly Ecosystem
Development Tools
-
Compilers and Toolchains:
wasm-pack
: Build Rust projects for Wasmemscripten
: Compile C/C++ code to WasmAssemblyScript
: Develop Wasm using TypeScript syntax
-
Debugging Tools:
- Chrome DevTools Wasm debugger
- Firefox WebAssembly debugger
wabt
: WebAssembly Binary Toolkit
WASI (WebAssembly System Interface)
WASI usage example:
// File system access using WASI
#[link(wasm_import_module = "wasi_snapshot_preview1")]
extern "C" {
fn fd_write(fd: u32, iovs_ptr: *const u8, iovs_len: u32, nwritten: *mut u32) -> u32;
}
Future Prospects
Future development directions for WebAssembly:
-
Component Model:
- Improved module dependency management
- Expanded package ecosystem
-
Garbage Collection Integration:
- Integration with JavaScript GC
- Reference counting optimization
-
Enhanced Threading Support:
- SharedArrayBuffer utilization
- Worker thread integration
-
New Use Cases:
- Serverless computing
- IoT devices
- Blockchain smart contracts
Conclusion
WebAssembly is transforming the future of web development, providing a powerful tool that offers both performance and security. Thanks to support across various languages and platforms, developers have the opportunity to deliver better user experiences.
References